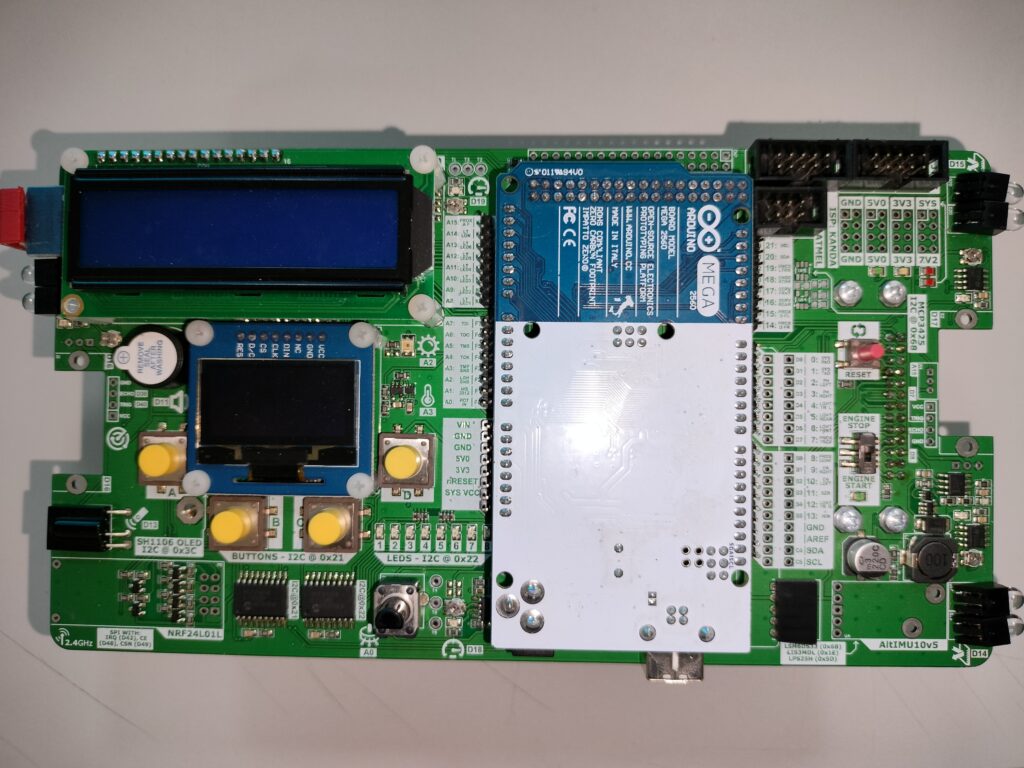
Documentation (Pinout) for Arduino Mega 2560: https://content.arduino.cc/assets/Pinout-Mega2560rev3_latest.pdf
Documentation: ATmega2560: https://ww1.microchip.com/downloads/en/devicedoc/atmel-2549-8-bit-avr-microcontroller-atmega640-1280-1281-2560-2561_datasheet.pdf
https://github.com/SKNMIPSA/IE-TRACK-KIT-PWR-1
Libraries for AVR: http://www.peterfleury.epizy.com/
Arduino | ATmega2560 | Description |
D9 | PH6 | gąsiennica prawa (uruchomienie) |
D10 | PB4 | gąsiennica lewa (uruchomienie) |
D2 | PE4 | kierunek gąsiennicy lewej |
D3 | PE5 | kierunek gąsiennicy prawej |
D6 | PH3 | światła przednie |
D5 | PE3 | światła tylne |
D14 | PJ1 | prawy przedni czujnik zbliżeniowy |
D15 | PJ0 | lewy przedni czujnik zbliżeniowy |
D36 | PC1 | tylni czujnik zbliżeniowy |
Microchip Studio configuration:
Commands to enter in Microchip Studio:
C:\Program Files (x86)\Arduino\hardware\tools\avr/bin/avrdude.exe
-C"C:\Program Files (x86)\Arduino\hardware\tools\avr/etc/avrdude.conf" -v -patmega2560 -cwiring -PCOM6 -b115200 -D -Uflash:w:"$(ProjectDir)Debug\$(TargetName).hex":i
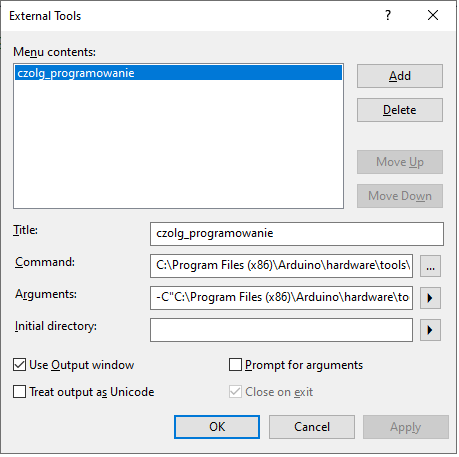
Configuration screen
Sample program
/*
* 2022_10_10_test.cpp
*
* Created: 10.10.2022 10:28:46
* Author : pskul
*/
#include <avr/io.h>
#define F_CPU 16000000UL
#include <util/delay.h>
int main(void)
{
//76543210
DDRH = 0b00001000;
PORTH = 0b00001000;
/* Replace with your application code */
while (1)
{
PORTH = 0b00001000;
_delay_ms(1000);
PORTH = 0b00000000;
_delay_ms(1000);
}
}
Another example
/*
* 2022_10_10_test.cpp
*
* Created: 10.10.2022 10:28:46
* Author : pskul
*/
#include <avr/io.h>
#define F_CPU 16000000UL
#include <util/delay.h>
int main(void)
{
//76543210
DDRH = 0b00001000;
PORTH = 0b00001000;
while (1)
{
PORTH |= (1<<3);//0b00001000;
_delay_ms(1000);
PORTH &= ~(1<<3);//~0b00001000;
_delay_ms(1000);
}
}
Timers and interrupts
/*
* Created: 16.10.2023 10:28:46
* Author : pskul @ chatGPT:)
*/
#include <avr/io.h>
#define F_CPU 16000000UL
#include <util/delay.h>
#include <avr/interrupt.h>
// Makrodefinicje dla ustawiania i resetowania bitów
#define SET_BIT(reg, bit) (reg |= (1 << bit))
#define CLEAR_BIT(reg, bit) (reg &= ~(1 << bit))
volatile int ledState = 0;
ISR(TIMER1_OVF_vect)
{
TCNT1 = 0xEFFF;
if (ledState==0) ledState = 1; else ledState = 0;
if (ledState==0)
{
PORTH &= ~(1<<3);
} else
{
PORTH |= (1<<3);//0b00001000;
}
}
int main(void)
{
//76543210
DDRH = 0b00001000;
PORTH = 0b00000000;
// Ustawienie preskalera na 1024
SET_BIT(TCCR1B, CS10);
CLEAR_BIT(TCCR1B, CS11);
SET_BIT(TCCR1B, CS12);
// Włączenie przerwań od przepełnienia licznika 1
SET_BIT(TIMSK1, TOIE1);
// Włączenie globalnych przerwań
sei();
while (1)
{
}
}
Preparing a report
The report should be prepared in the English language and include the authors’ names along with their student ID numbers.
It should begin with an introductory paragraph, providing a brief description of what was accomplished within the project.
Following that, list the components used in the project (e.g., Bluetooth module, LCD display) and justify their utilization in the project. The next section should detail any external libraries utilized, along with references to their online sources.
In the documentation, please include a photo of auxiliary applications (e.g., mobile app) as well as a photo of the functioning circuit (to show messages on the display, lit LEDs, etc.).
Source code should be embedded within the document (not sent as separate files) in the format: filename, source code. All variables and comments within the code must be in the English language.
After demonstrating the project’s functionality, please send the PDF document to the instructor’s email address. The email content should be composed in English.